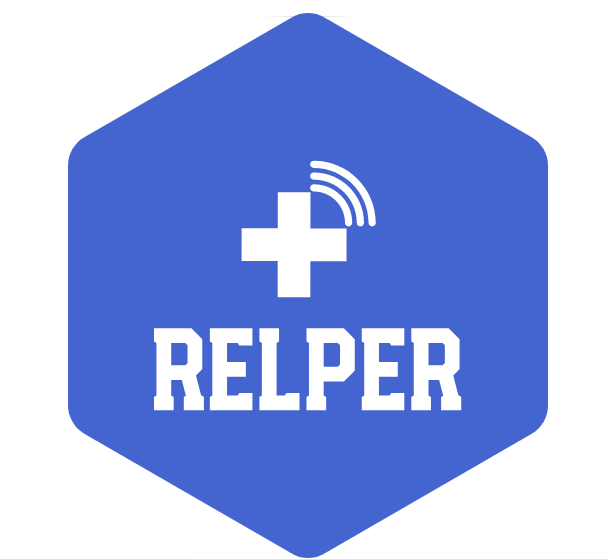
Functions is_
functions_is.Rmd
is_
functions returns a boolean, given a condition.
is_datetime
The goal of is_datetime
is to check if a value is a
datetime.
is_datetime(Sys.time())
#> [1] TRUE
is_datetime(Sys.Date())
#> [1] FALSE
is_integer
The goal of is_integer
is to check if a value is a
integer, not considering their variable type but the value.
is_integer(1.1)
#> [1] FALSE
is_integer(1)
#> [1] TRUE
is_negative
The goal of is_negative
is to check if a value is
negative.
is_negative(1)
#> [1] FALSE
is_negative(-1)
#> [1] TRUE
is_outlier
The goal of is_outlier
is to check if a value is an
outlier, by using the boxplot outlier criteria, given by:
where:
- is the first quartile;
- is the third quartile;
- is the interquartile range, e.g., .
x <- c(1,2,3,5,7,8,12,100)
is_outlier(x)
#> [1] FALSE FALSE FALSE FALSE FALSE FALSE FALSE TRUE
is_positive
The goal of is_positive
is to check if a value is
positive.
is_positive(1)
#> [1] TRUE
is_positive(-1)
#> [1] FALSE
is_weekend
The goal of is_weekend
is to check if a date variable is
in a weekend (saturday/sunday).
is_weekend(Sys.time())
#> [1] FALSE
is_weekend(Sys.Date())
#> [1] FALSE